1.18.1.5.1 Simple Hello World Dialogfirst-HTML-dialog
Hello World DialogHTML Dialog
Summary
This tutorial will show how to:
- Create an HTML page.
- Display the HTML page in the dialog box by OriginC.
- Build an event handler to respond to events of dialog.
Minimum Origin Version Required: Origin 2017
Sample Files
- index.html: This is the HTML code for the html page.
- HelloWorldDlg.cpp: This is the OriginC code to call out the HTML page and show it in the dialog.
- HelloWorldDlg1.cpp: This is the OriginC code that includes more advanced features.
Note: You can download the sample files here.
|
Creating an HTML Page
When you create an HTML dialog, the first step is creating an HTML page.
- Start Origin and open Code Builder by clicking button
.
- Create a new HTML file named index.html and save it to a new folder named Hello World.
- Inside the< head > and < body > elements, add the other HTML elements such as < title >, < h1 >, < p > to construct an HTML page.
<!DOCTYPE html>
<html>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=Edge"/>
<head>
<title>
A Small Hello
</title>
</head>
<body>
<h1>Hello World</h1>
<p>This is very minimal "hello world" HTML document.</p>
</body>
</html>
Note: When editing is complete, you can check the content of this page by opening it with any web browser.
|
Creating an HTML dialog
In this section, you will make the generated HTML page display in the dialog.
- In Code Builder, create a new cpp file named HelloWorldDlg.cpp under the path of the HTML file.
- Include three needed header files at first. Note that HTMLDlg.h is new in Origin 2017 which connects Origin and the HTML dialog together.
#include <Origin.h>
#include <..\OriginLab\DialogEx.h>
#include <..\OriginLab\HTMLDlg.h>
- Derive a user defined HTML dialog class from the class
HTMLDlg , and point to the HTML page inside the method GetInitURL() .
class HelloWorldDlg: public HTMLDlg
{
public:
string GetInitURL() //get the path of html file
{
string strFile = __FILE__; //the path of the current file
return GetFilePath(strFile) + "index.html";
}
};
- To open the HTML dialog, save all the code and build it, then execute the following function.
void hello()
{
HelloWorldDlg dlg;
dlg.DoModalEx(GetWindow());
}
An HTML dialog with "Hello World" will pop up:
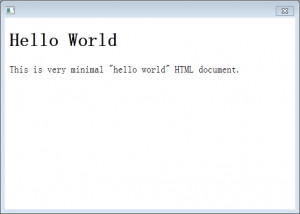
Advanced Feature
If you want to learn more, try to improve the program by modifying the dialog and adding event handlers.
Note: The complete code of the following features is available in HelloWorldDlg1.cpp.
|
Preventing dialog resizing
To do this, use ModifyStyle to disable WS_THICKFRAME in the method OnInitDialog inside your dialog class:
BOOL OnInitDialog()
{
ModifyStyle(WS_THICKFRAME, 0);//prevent resizing
return HTMLDlg::OnInitDialog();
}
Setting dialog size
You need to call a parent class method GetDlgInitSize from you dialog class, and specify the width and height of the dialog in it:
BOOL GetDlgInitSize(int& width, int& height)//get dialog size
{
width = 500;
height = 200;
return true;
}
Adding event handler
Similar to Dialog Builder, a message map is used to specify which events will be handled and which function will be called to handle them.
As an example, an event handler methods are added inside the user defined class HelloWorldDlg to pop up a message when the dialog is closed.
- Map the dialog message by the following message map inside the class
HelloWorldDlg .
//Message Map
public:
EVENTS_BEGIN_DERIV(HTMLDlg)
ON_DESTROY(OnDestroy)
EVENTS_END_DERIV
- Add an event handler method inside the class
HelloWorldDlg to pop up a message box when the dialog is closed.
BOOL OnDestroy()
{
MessageBox(GetSafeHwnd(), _L("Thank you and have a good day!") ,_L("Message"));
return true;
}
|