5.6 Debug LabTalk ScriptTutorial-Debug
Summary
Origin provides various tools to help you to develop and debug your LabTalk scripts.
What You Will Learn
This tutorial will show you how to:
- Debug LabTalk script by using Code Builder.
- Debug LabTalk script by using some useful skills.
- Handle the error to make LabTalk script running without interruption.
- Understand the concepts of LabTalk script precedence.
Using Code Builder
Once you save the LabTalk script in a section of a text file with *.OGS type, you can use Code Builder to debug the LabTalk script.
Following is an example that show you how to use Code Buider to do debugging.
- Open the *.ogs file in Code Builder.
- Set a breakpoint to a line by moving the cursor to that line and pressing F9 (or selecting Debug:Toggle Breakpoints from the menu, or clicking the Toggle Breakpoint button
), or just click on the margin to the left of the line number.
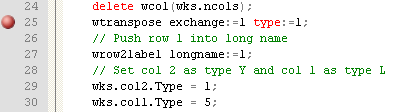
- Run the section. You can select the current section name or just put the cursor to the line of section name, and then click the Execute Current Section button
or select Debug:Execute Current Section from the menu to run up the code to the specified line. Also, you can use the run.section object method to run the section.
- Click the Step Into button
(or press F8, or select Debug: Step Into from menu) to execute the script step by step to check the logic error.
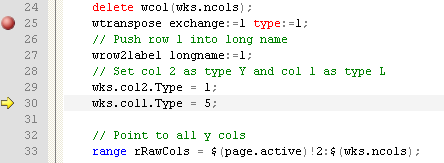
- If there is another breakpoint, you can click the Go button
to run up the script to the next breakpoint. After you finish the debugging, click the Stop Debugging button to end up the debugging.
If there is a command line calling an LabTalk function, LabTalk callable OC function, another section in the same ogs or different ogs, you can choose if execute the code one line at a time including the called function (or called section, called ogs).
Step Into
The Step Into feature lets you step through a called function to check for logic errors in the function.
- Set a breakpoint at the line that calls getminmax() function.
- Step through your code line by line until you reach the function call. While the program is paused at the function call line, click the Step Into button
on the Debug toolbar or select Debug:Step Into.
- The debugger executes the function call statement and then pauses execution at the first line of the called section.
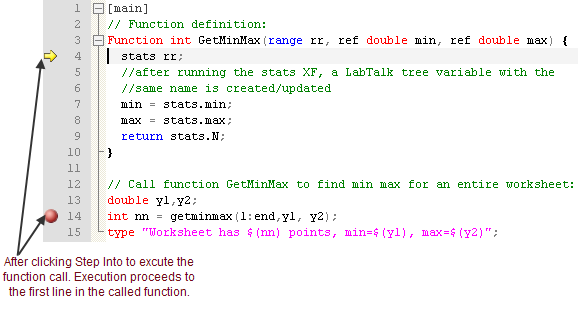
Step Over
However, you can also step over a called function. To do this, pause the code execution at the function call line that you want to step over (either by setting a breakpoint or by executing line by line up to the function call). While the program is paused at the function call line, click the Step Over button on the Debug toolbar or select Debug:Step Over.
The debugger executes the function call and then pauses after the function returns.
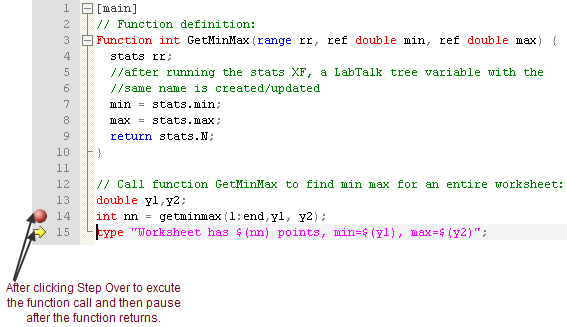
Step Out
When execution is paused inside a function, you can click the Step Out button on the Debug toolbar or select Debug:Step Out to step out of the function.
Run to Cursor
If no breakpoint interrupts the routine, click the Run to Cursor button or select Debug: Run to Cursor, the script will run until it reaches the location where the cursor is set.
Additionally, you can open a labtalk script in code builder by the ed.open() method.
For example, to open the specified script file at the specified section in a Code Builder window, you can write script as below.
cd 2; //Switch to the labtalk sacript sample folder of Origin
ed.open(summarize_data.ogs,main);
Using Skills
win -o; layer -o
Although the win -o and layer -o command s aren't related to debugging, they are very useful to avoid errors from happenning.
When working on an Origin Object, like a workbook or graph page/layer, a script always operates on the active window by default. But sometimes, you don't want to activate the target window as switching active windows or layers may have delay and may lead to unpredictable outcome. In this case, you'd better to use win -o or layer -o to enclose the script.
newbook; //new a workbook.
//import file to workbook.
string fn1$=system.path.program$ + "Samples\Spectroscopy\XPS\BTABA.DAT";
impasc fname:=fn1$;
string mybk$=%H; //save the workbook name
plotxy (1,2) o:=<new>; //create a graph, the graph window is active.
//run the script in curly brace for the workbook window.
win -o mybk$
{
newsheet;
string fn2$=system.path.program$ + "Samples\Spectroscopy\XPS\BTATI.DAT";
impasc fname:=fn2$;
plotxy (1,2) o:=<new>;
}
type
Type can be use to print out the contents of the specified script file (.OGS file) in the current directory to the Command (or Script) window.
For example,
cd 2;
type autofit; //print the labtalk example file autofot.ogs.
echo
To debug and trace, the system variable, Echo prints scripts or error messages to the Command window (or Script window when it is entered there).
For example, run the follwoing script in Script window.
echo=1;
string aa$="abc";
double aa=100;
Not only the general error meaage will show, but also the script line with error.
AA:already declared as string, cannot redeclare in the same scope!
Error:double aa=100 //The script line with error will show after runing echo=1.
Error Handling
When there is an error in your LabTalk code, the code will be interrupted at the point of the error and not execute any lines after the error. If you want the script to continue executing without interruption, you can consider using curly braces around the line(s) with error(s).
For instance, run the following script,
newbook name:=abc option:=lsname;
wks.ncols=3;
col(1)=data(1,10);
col(2)=normal(10);
plotxy;
win -a abc;
col(3)=col(1)+col(2);
Col(1) and Col(2) will be filled with data, but the script will stop executing at the line "plotxy;" as no input has been specified. So Col(3) will not be filled.
If you surround the line "plotxy;" with curly braces as below,
newbook name:=abc option:=lsname;
wks.ncols=3;
col(1)=data(1,10);
col(2)=normal(10);
{plotxy;}
win -a abc;
col(3)=col(1)+col(2);
the next line will execute and Col(3) will be filled.
For more details about error handling, you can read this page.
LabTalk Script Precedence
When a user-defined Origin C function has same name as a labtalk ogs file, the labtalk ogs file has higher precedence.
For instance, create an Origin C function, "precedence",
void precedence()
{
MessageBox(GetWindow(), "This message is from OC code!", "OC");
}
Compile this Origin C function and then run
precedence;
in the Script window. A message box will pop up saying "This message is from OC code!".
Then create an ogs file, name it "precedence.ogs", and save it in the User File folder with the following script:
type -b "This message is from OGS file!";
After that, run the below script in Script window:
cd 1; // current working directory
precedence;
A message box will appear saying "This message is from OGS file!". It illustrates the labtalk script has higher precedence.
|