5 Supporting Multiple Clients with OriginPro
OriginPro supports two additional methods that can be used to lock an existing instance of Origin to one client, thereby denying access to other clients until the current client releases the connection (with Origin):
- The BeginSession method reserves the current Origin session for exclusive use by a client.
- The EndSession method releases the current Origin session for use by other clients.
These two methods have meaning only for clients accessing a single instance of the Origin automation server. In other words, the clients need to declare the Origin object using the ApplicationSI or ApplicationCOMSI methods. The BeginSession and EndSession methods are available only to clients connecting to an OriginPro session.
The following example show how Session works in OriginPro.
Origin Session Demo
This example is created in Visual Studio 2005, with C#.
- The first step is to create a simple C# Window Application, with name "OriginSession".
- Turn to resource view and add buttons on the dialog, and a Text label and a Timer control to show time elapse during a session, like the following picture shows:
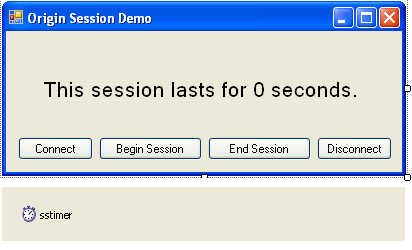
- Add Origin.ApplicationSI m_pOrigin and an integer nSeconds as the Form class's members.
- Add code to handle events when button clicked:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace OriginSession
{
public partial class MainForm : Form
{
private Origin.ApplicationSI m_pOrigin;
private int nSeconds = 0;
public MainForm()
{
InitializeComponent();
}
private void Connect_Click(object sender, EventArgs e)
{
try
{
m_pOrigin = new Origin.ApplicationSIClass();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
return;
}
try
{
if (m_pOrigin != null)
m_pOrigin.Visible = Origin.MAINWND_VISIBLE.MAINWND_SHOW;
}
catch (Exception ex2)
{
MessageBox.Show(ex2.Message);
return;
}
Connect.Enabled = false;
Disconnect.Enabled = true;
BeginSession.Enabled = true;
}
private void MainForm_Load(object sender, EventArgs e)
{
sstimer.Enabled = false;
Connect.Enabled = true;
Disconnect.Enabled = false;
BeginSession.Enabled = false;
EndSession.Enabled = false;
nSeconds = 0;
}
private void BeginSession_Click(object sender, EventArgs e)
{
bool bSuccess = false;
try
{
bSuccess = m_pOrigin.BeginSession();
}
catch (Exception ex1)
{
MessageBox.Show(ex1.Message);
return;
}
if ( !bSuccess )
{
MessageBox.Show("Can not begin Origin session, maybe it is possessed by another client!");
return;
}
sstimer.Enabled = true;
EndSession.Enabled = true;
BeginSession.Enabled = false;
Disconnect.Enabled = false;
}
private void EndSession_Click(object sender, EventArgs e)
{
bool bSuccess = m_pOrigin.EndSession();
if (!bSuccess)
{
MessageBox.Show("Fail to end Origin session!");
}
nSeconds = 0;
sstimer.Enabled = false;
BeginSession.Enabled = true;
EndSession.Enabled = false;
Disconnect.Enabled = true;
string strMsg = "This session lasts for " + (nSeconds) + " seconds.";
SessionTimer.Text = strMsg;
}
private void Disconnect_Click(object sender, EventArgs e)
{
if (m_pOrigin != null)
{
System.Runtime.InteropServices.Marshal.FinalReleaseComObject(m_pOrigin);
m_pOrigin = null;
Disconnect.Enabled = false;
Connect.Enabled = true;
BeginSession.Enabled = false;
EndSession.Enabled = false;
string strMsg = "This session lasts for " + (nSeconds) + " seconds.";
SessionTimer.Text = strMsg;
}
}
private void sstimer_Tick(object sender, EventArgs e)
{
string strMsg = "This session lasts for " + (++nSeconds) + " seconds.";
SessionTimer.Text = strMsg;
}
}
}
When run this example, please launch multiple instance of the dialog application, click Connect and Begin Session on one of these dialogs, then try the same steps on any other instance will cause exception until End Session clicked on the first dialog, since during a session, Origin is possessed exclusively by the first instance.
Several examples of client applications connecting to Origin from Visual Basic, Visual C++, Excel, MATLAB, and LabVIEW can be found in the subfolder \Samples\COM Server and Client\ under the main Origin installation folder. See Automation Server Examplesfor complete examples.
|